Next.jsにJestを入れたい
この記事は最終更新日から1年以上が経過しています。

Next.jsのプロジェクトにJestをインストールした時のことをメモしました。
ざっくりいうとこちら(https://nextjs.org/docs/testing#setting-up-jest-with-the-rust-compiler)を参考にインストールしました!
まずはコマンドを叩きます。
$ npm install --save-dev jest @testing-library/react @testing-library/jest-dom react-test-renderer
プロジェクトのルートディレクトリにjest.config.js
ファイルを作成し、以下を追加します。
// jest.config.js
const nextJest = require('next/jest')
const createJestConfig = nextJest({
// Provide the path to your Next.js app to load next.config.js and .env files in your test environment
dir: './',
})
// Add any custom config to be passed to Jest
const customJestConfig = {
setupFilesAfterEnv: ['<rootDir>/jest.setup.js'],
}
// createJestConfig is exported this way to ensure that next/jest can load the Next.js config which is async
module.exports = createJestConfig(customJestConfig)
そしてpackage.jsonの'scripts'
に追記します。
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"test": "jest --watch" // 追記しました。
}
それではtestファイルを作成します。
プロジェクトのルートディレクトリに__tests__フォルダーを作り
テストを追加します。
↓ __tests__/index.test.tsx
/**
* @jest-environment jsdom
*/
import React from 'react'
import { render, screen } from '@testing-library/react'
import Home from '../pages/index'
describe('Home', () => {
it('renders a heading', () => {
render(<Home />)
const heading = screen.getByRole('heading', {
name: /welcome to next\.js!/i,
})
expect(heading).toBeInTheDocument()
})
})
スナップショットテストも追加します。
↓ __tests__/snapshot.tsx
import React from 'react'
import renderer from 'react-test-renderer'
import Index from '../pages/index'
it('renders homepage unchanged', () => {
const tree = renderer.create(<Index />).toJSON()
expect(tree).toMatchSnapshot()
})
TypeScriptで動かしたいので、こちらを追加します。
$ npm i --save-dev @types/jest
そしてtsconfig.jsonのcompilerOptionsのtypesに下記をを追加します。
"types": ["jest", "@testing-library/jest-dom"], // 追記しました
では、実行します。
$ npm run test
!
エラーでした!
jest.setup.jsにsetupFilesAfterEnvを追加してくださいとのようです。

jest.setup.jsを作成しました。
// jest.setup.js
import '@testing-library/jest-dom/extend-expect'
ではtestします。
$ npm run test
お!
実行できました。
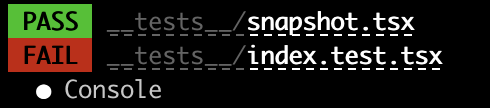
index.test.tsxはテスト通りませんでしたが、無事にJestは動きました。
ここまでお読みいただきありがとうございました。